When a customer placed an order via the Stripe WooCommerce Extension, you may want to send some of those details to Stripe as metadata:
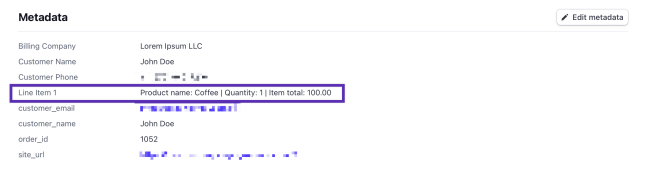
To do this, you can leverage the wc_stripe_payment_metadata
filter.
NOTE: We are unable to provide support for custom code under our Support Policy. If you need to customize a snippet further or extend its functionality, we highly recommend Codeable or a Certified WooExpert.
For example, to send customer, order, and product data to Stripe as metadata, you can use this snippet:
/**
Add Stripe metadata along with WooCommerce purchase
*
@param $metadata
@param $order
@param $source
@return mixed
*/
function customstripepayment_metadata( $metadata, $order, $source ) {
/**
* Get order data
*/
$order_data = $order->get_data();
$metadata[ __( 'Billing Company', 'woocommerce-gateway-stripe' ) ] = sanitize_text_field( $order_data['billing']['company'] );
$metadata[ __( 'Customer Name', 'woocommerce-gateway-stripe' ) ] = sanitize_text_field( $order_data['billing']['first_name'] . ' ' . $order_data['billing']['last_name'] );
$metadata[ __( 'Customer Phone', 'woocommerce-gateway-stripe' ) ] = sanitize_text_field( $order_data['billing']['phone'] );
/**
* List products purchased
*/
$count = 1;
foreach( $order->get_items() as $item_id => $line_item ){
$product = $line_item->get_product();
$product_name = $product->get_name();
$item_quantity = $line_item->get_quantity();
$item_total = $line_item->get_total();
$metadata['Line Item '.$count] = 'Product name: '.$product_name.' | Quantity: '.$item_quantity.' | Item total: '. number_format( $item_total, 2 );
$count += 1;
}
return $metadata;
}
add_filter( 'wc_stripe_payment_metadata', 'customstripepayment_metadata', 10, 3 );